More obvious questions from the brat with the usual number of toes:
I write web software using asp.net. I have a “web page” that takes ?number=x in the url and saves the number in a database for no particular purpose. You can add numbers to my database all you like at:
http://barefootelectronics.com/particleposttest.aspx?number=222
(Until I kill it because I want to make something useful)
I don’t have a page to show you the numbers, but I see the numbers and times in the table in my database.
So I made a web hook to try and put a number from my particle electron. I think I have a screen shot at
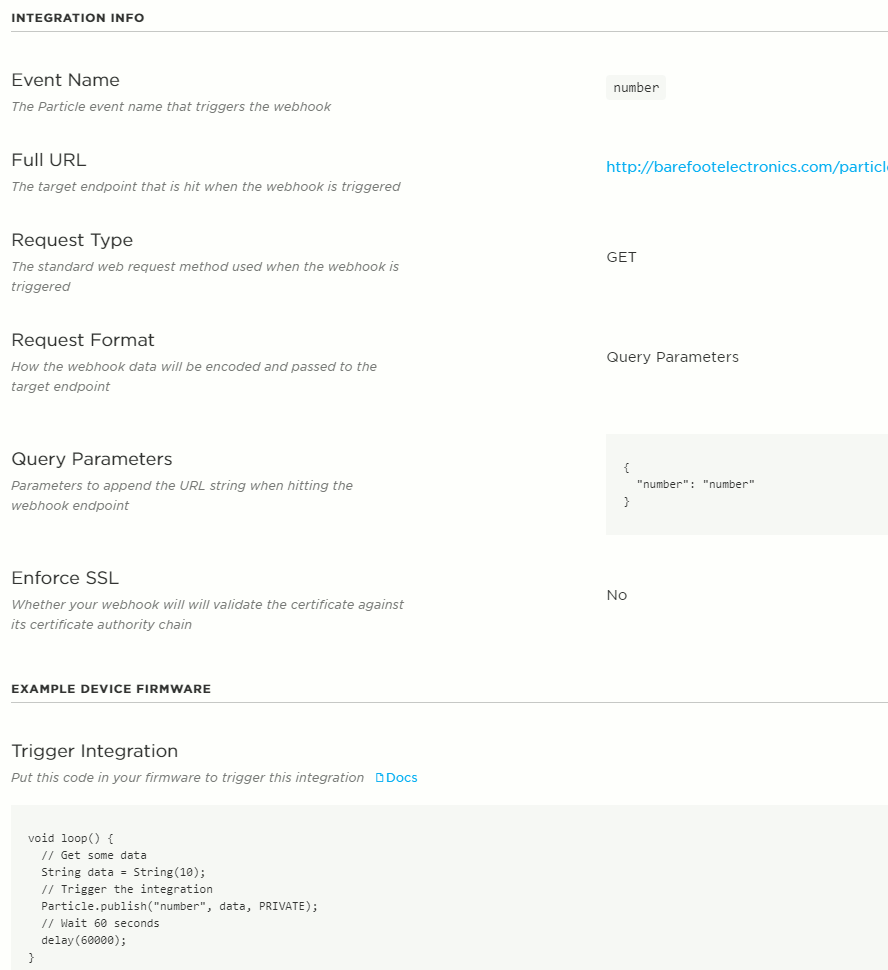
And I made my program:
// -----------------------------------
// Controlling LEDs over the Internet
// -----------------------------------
// First, let's create our "shorthand" for the pins
// Same as in the Blink an LED example:
// led1 is D0, led2 is D7
int led1 = D6;
int led2 = D7;
// Last time, we only needed to declare pins in the setup function.
// This time, we are also going to register our Particle function
int photoresistor = A0; // This is where your photoresistor is plugged in. The other side goes to the "power" pin (below).
int power = A5;
int analogvalue;
void setup()
{
// Here's the pin configuration, same as last time
pinMode(led1, OUTPUT);
pinMode(led2, OUTPUT);
// We are also going to declare a Particle.function so that we can turn the LED on and off from the cloud.
Particle.function("led",ledToggle);
// This is saying that when we ask the cloud for the function "led", it will employ the function ledToggle() from this app.
// For good measure, let's also make sure both LEDs are off when we start:
digitalWrite(led1, LOW);
digitalWrite(led2, LOW);
pinMode(photoresistor,INPUT); // Our photoresistor pin is input (reading the photoresistor)
pinMode(power,OUTPUT); // The pin powering the photoresistor is output (sending out consistent power)
// Next, write the power of the photoresistor to be the maximum possible, so that we can use this for power.
digitalWrite(power,HIGH);
// We are going to declare a Particle.variable() here so that we can access the value of the photoresistor from the cloud.
Particle.variable("analogvalue", &analogvalue, INT);
}
// Last time, we wanted to continously blink the LED on and off
// Since we're waiting for input through the cloud this time,
// we don't actually need to put anything in the loop
unsigned long timewas = 0 ;
void loop()
{
unsigned long timeis = millis();
if ((timeis % 1000) > 500)
{
digitalWrite(led2,HIGH);
}
else
{
digitalWrite(led2,LOW);
}
analogvalue = analogRead(photoresistor);
if ((timeis/1000) > timewas )
{
timewas = timeis/1000;
String temp = String(analogvalue);
Particle.publish("number", temp, PRIVATE);
}
}
// We're going to have a super cool function now that gets called when a matching API request is sent
// This is the ledToggle function we registered to the "led" Particle.function earlier.
int ledToggle(String command) {
/* Particle.functions always take a string as an argument and return an integer.
Since we can pass a string, it means that we can give the program commands on how the function should be used.
In this case, telling the function "on" will turn the LED on and telling it "off" will turn the LED off.
Then, the function returns a value to us to let us know what happened.
In this case, it will return 1 for the LEDs turning on, 0 for the LEDs turning off,
and -1 if we received a totally bogus command that didn't do anything to the LEDs.
*/
if (command=="on") {
digitalWrite(led1,HIGH);
return 1;
}
else if (command=="off") {
digitalWrite(led1,LOW);
return 0;
}
else {
return -1;
}
}
You see in my loop, I particle.publish to my “number” hook every second. I can turn the other LED on and off with the function, and I can see the light level in the variable, but I don’t get entries in my useless table from it getting the url… So obviously, I have my web hook wonky or my logic isn’t actually posting it every second.