n00b to Particle and while I’ve had some limited experience with development in general, safe to same it’s been more tweaking other examples that learning things from the ground up.
I’m working on a webhook & app to light up LEDs in my Photon based on bus arrival data from the Transport for London (tfl) API. I’m basing the project on @LukeUSMC’s awesome example here: Tutorial: Webhooks and Responses with Parsing (JSON, Mustache, Tokens)
I’ve got the webhook running (not elegant, but that’s another story) and now trying to build out the rest of the application for my needs.
I’m running into an issue very early on - this not uncommon ‘xxx does not name a type’ error. I’ve seen a few people solving it via #include “application.h” - but doesn’t do the trick for me. So, my current setup:
A single .ino file (at the end of the thread if interested), where I’m defining the hook and response, the subscribe and publish and eventually what the LEDs should do based on the response.
However I get stuck why trying to verify the code very early on. A few thoughts:
- Could be an issue with needing to have .ino and .cpp file split (the error messages have my .ino file name shown as .cpp, so don’t fully understand that).
- Could be a simple issue with my code
- Could be some other obvious error (misplacement of things in the setup {} vs loop{} etc.
The error (as well as a second one, but I’ll deal with that later unless you want to chime in)
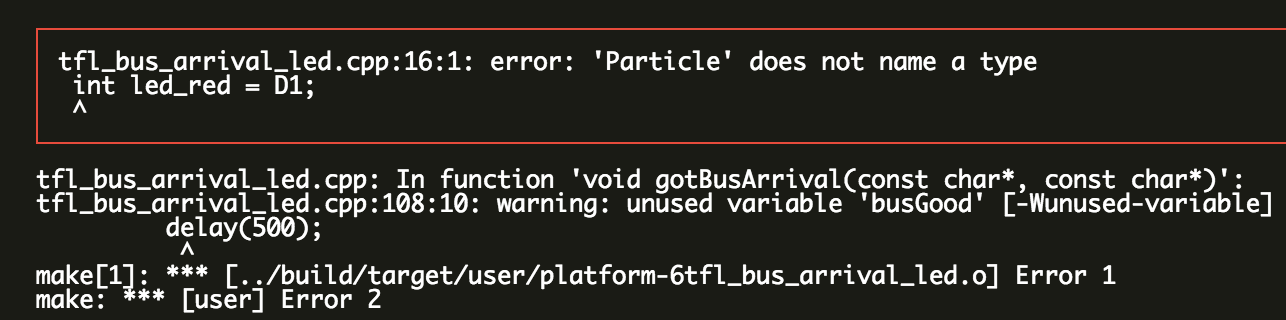
The lines of my code ending in the line throwing the error:
#define HOOK_RESP "hook-response/tfl274_hook_3"
#define HOOK_PUB "tfl274_hook_3"
int led_red = D1;
int led_default = D7;
int busGood;
int badBusCall;
int updatebusminute;
//This will call the function gotBusArrivalData when the particle.io cloud posts a hook response that matches my HOOK_RESP in my definitions.
Particle.subscribe(HOOK_RESP, gotBusArrivalData, MY_DEVICES);
Screenshot, as I’m a little worried about the / at the top and the color going from purple to white as it could be a problem - but maybe unrelated.
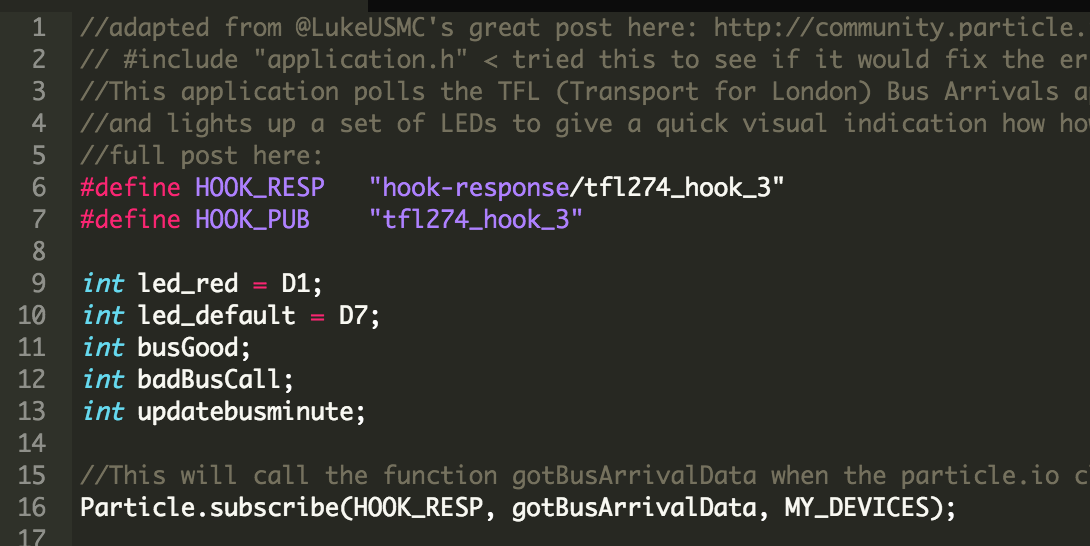
Full code here:
//adapted from @LukeUSMC's great post here: http://community.particle.io/t/execute-function-once-a-day-or-after-reset-reboot/14521
// #include "application.h" < tried this to see if it would fix the error, it didn't
//This application polls the TFL (Transport for London) Bus Arrivals app for a given Bus Stop
//and lights up a set of LEDs to give a quick visual indication how how much time you have
//full post here:
#define HOOK_RESP "hook-response/tfl274_hook_3"
#define HOOK_PUB "tfl274_hook_3"
int led_red = D1;
int led_default = D7;
int busGood;
int badBusCall;
int updatebusminute;
//This will call the function gotBusArrivalData when the particle.io cloud posts a hook response that matches my HOOK_RESP in my definitions.
Particle.subscribe(HOOK_RESP, gotBusArrivalData, MY_DEVICES);
//Now lets trigger (Spark.publish) our webhook in our code so the particle.io cloud will
//reach out to the API we designated and post a response. In my instance the call looks like this:
// Spark.publish(HOOK_PUB);
//This actually isn't sufficient though. I have learned that you need to give the cloud some time to respond or
//depending on how you are calling this your loop will post your Spark.publish over and over. Here is my getWeather
//function (which is a slightly modified version of @peekay123 getWeather function in his RGB Pong Clock project Peekay123 RGBPong Clock Github5).
//Updates Bus Arrival Data
void getBusArrival() {
busGood = false;
Particle.publish(HOOK_PUB);
unsigned long wait = millis();
while (!busGood && (millis() < wait + 5000UL))
Particle.process();
if (!busGood) {
badBusCall++;
if (badBusCall > 2) {
}
}
else
badBusCall = 0;
}
// Now we've built a webhook, subscribed to that event and have called for particle.io to give us the response
//which will in turn call our gotBusArrival function. The gotBusArrival function will parse the response from a
//bunch of numbers with ~ between them to the data I actually want. Here is my gotBusArrival function which uses
//the ~ to tokenize the data and maps it to int variables for use elsewhere.
void gotBusArrival(const char *name, const char *data) {
String str = String(data);
char strBuffer[125] = "";
str.toCharArray(strBuffer, 125); // example: "\"21~99~75~0~22~98~77~20~23~97~74~10~24~94~72~10~\""
//TODO deal with consecutive ~ chars - what happens?
//TODO deal with up to 10 busses arriving - array perhaps? Below is ugly.
int arrivalsec1 = atoi(strtok(strBuffer, "\"~"));
int arrivalroute1 = atoi(strtok(NULL, "~"));
//then values for the second bus
int arrivalsec2 = atoi(strtok(NULL, "~"));
int arrivalroute2 = atoi(strtok(NULL, "~"));
//then values for the third bus
int arrivalsec3 = atoi(strtok(NULL, "~"));
int arrivalroute3 = atoi(strtok(NULL, "~"));
//then values for the fourth bus
int arrivalsec4 = atoi(strtok(NULL, "~"));
int arrivalroute4 = atoi(strtok(NULL, "~"));
//then values for the third bus
int arrivalsec5 = atoi(strtok(NULL, "~"));
int arrivalroute5 = atoi(strtok(NULL, "~"));
//Set the LED to blink slowly if bus arriving in less than 5min
//otherwise off
if ((arrivalsec1/60 <5 && arrivalroute1 == 274 ) or (arrivalsec2/60 <5 && arrivalroute2 == 274 ) or (arrivalsec3/60<5 && arrivalroute3 == 274 ) or (arrivalsec4/60<5 && arrivalroute4 == 274 ) or (arrivalsec5/60<5 && arrivalroute5 == 274 ) ){
// To blink the LED, first we'll turn it on...
digitalWrite(led_red, HIGH);
// We'll leave it on for 1 second...
delay(1000);
// Then we'll turn it off...
digitalWrite(led_red, LOW);
// Wait 1 second...
delay(1000);
}
//Set the second LED to blink more quickly if less than 2 min
else if ((arrivalsec1/60 <2) or (arrivalsec2/60 <2) or (arrivalsec3/60<2) or (arrivalsec4/60<2) or (arrivalsec5/60<2)){
// To blink the LED, first we'll turn it on...
digitalWrite(led_default, HIGH);
delay(500);
digitalWrite(led_default, LOW);
delay(500);
}
else {
digitalWrite(led_red, LOW);
digitalWrite(led_default, LOW);
}
bool busGood = true;
updatebusminute = Time.minute();
}
void setup() {
pinMode(led_red, OUTPUT);
pinMode(led_default, OUTPUT);
}
void loop() {
if (Time.minute() != updatebusminute){
getBusArrival();
}
}